Handling API response in React
Handling API response in React
Working with React to build a web application, one of the most common things to practice is accessing responses from REST APIS. Most of the applications which are based on real time have backends working with API calls. In this, the web page fetches data from an endpoint server and renders them accordingly.
For instance, if you are making a website for social media, and you want to display stored images, statuses, and other things. You need to call the API and get all the data, save them, and render them accordingly.
Pre-requisite
The first thing to do is setting up React environment.
Use this code
npx create-react-app api-testing
This will download and set up the React environment for development. Next is that we need to install one more library to make API calls. That is AXIOS.
npm install axios
There are many endpoints that are free, available on the internet. For this, I will show an example with the base URL: https://api.themoviedb.org/3/.
This has its personal key which you can find after log in. The main purpose of this URL is to provide a database of movies in a sorted manner. Like trending movies, series, and more.
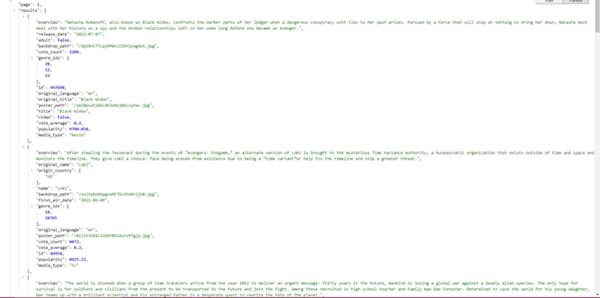
As you can see that you can get many things from API calls. Make sure to read the documentation in order to understand the functioning of the URL.
Storing responses and creating states
Now that you have the URL and you know what it is giving back as a response, it's time to store them locally so that they could be rendered.
To start with it first import useState and Axios from React and axios respective.
The useState is used to create a state to have a piece of retrieved response saved.
import React, { useState } from 'react'
Now create a state variable of let’s say movies and for this create a hook to set the movies named setMovies.
const [movies, setMovies] = useState([]);
Since all the movies will come in an array we will make it as an array only.
A simple hooks implementation can be carried out by firing an asynchronous function using the async keyword inside useEffect() and passing an empty array as a parameter.
Inside the useEffect we can use the axios to fetch the data.
Put the following code to import axios
import axios from './axios'
Inside the useEffect you can either call another function or create an async function to call the API.
Code:
useEffect(() =>{
// if [] run once when the row loads and don't run again
async function fetchData() {
const request = await axios.get(https://api.themoviedb.org/3//trending/all/week?api_key=xxxXXXKEYXXXxxx&language=en-US)
setMovies(request.data.results)
return request;
}
fetchData();
}, [fetchUrl])
Explanation:
In the above code, there is a function of fetchData which is calling API of given url by using axios, the response is then stored in a variable with the help of await keyword. Later on, when the data is retrieved the state is changed by setMovies, and the data is saved is then returned.
At last, you have to understand that using APIS is very convenient. The more you work upon it the more you will get clear how to access the data, by using various functionalities such as mapping and using indices. You can also interact with APIS in different ways like not only to get data by also to post data by using POST. This will add data from the user side to the database.