Xamarin.Forms - Debugging WebView
Introduction
Xamarin.Forms code runs on multiple platforms, each of which has its own filesystem. This means that reading and writing files are most easily done using the native file APIs on each platform. Alternatively, embedded resources are a simpler solution to distribute data files with an app.
Debugging Webview
Mobile developers are facing major difficulties in debugging the web URLs. I going to explain how to debug your xamarin.forms webview in an Emulator or simulator.
Prerequisites
- Visual Studio 2017 or later (Windows or Mac)
Setting up a Xamarin.Forms Project
Start by creating a new Xamarin.Forms project. You will learn more by going through the steps yourself.
Create a new or existing Xamarin forms (.Net standard) Project with Android and iOS Platform.
Choose the Mobile App (Xamarin. forms) project under C# and Mobile.
Name your app. You probably want your project and solution to use the same name as your app. Put it on your preferred location for projects and click "Create".
Now, select the blank app and target platforms - Android, iOS and Windows (UWP).
Subsequently, go to the solution. In there, you get all the files and sources of your project (.NET Standard). Now, select the XAML page and double-click to open the MainPage.Xaml page.
You now have a basic Xamarin.Forms app. Click the Play button to try it out.
Create a Custom Webview
Here going to create a custom webview inherit from webview for debug webview.
CustomWebView.cs
public class CustomWebView : WebView
{
}
Android Implementation
Here, Create a custom renderer for enable debugging in android.
CustomWebViewRenderer.cs
[assembly: ExportRenderer(typeof(CustomWebView), typeof(CustomWebViewRenderer))]
namespace XamWebView.Droid
{
public class CustomWebViewRenderer:WebViewRenderer
{
public CustomWebViewRenderer(Context context) : base(context)
{
}
protected override void OnElementChanged(ElementChangedEventArgs<WebView> e)
{
base.OnElementChanged(e);
global::Android.Webkit.WebView.SetWebContentsDebuggingEnabled(true);
}
}
}
iOS Implementation
Here, Create a custom renderer for enabling the Debugging in Webview.
CustomWebViewRenderer.cs
[assembly: ExportRenderer(typeof(CustomWebView), typeof(CustomWebViewRenderer))]
namespace PDFPOC.iOS
{
public class CustomWebViewRenderer : WebViewRenderer
{
protected override void OnElementChanged(VisualElementChangedEventArgs e)
{
base.OnElementChanged(e);
if (NativeView != null && e.NewElement != null)
{
var webView = NativeView as UIWebView;
if (webView == null)
return;
webView.ScalesPageToFit = true;
}
}
}
}
Consuming the CustomWebview
Here, consume the Custom Webview in your XAML
Add Namespace
xmlns:controls="clr-namespace:XamWebView"
Add your Custom Webview
MyWebPage.xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
xmlns:controls="clr-namespace:XamWebView"
x:Class="XamWebView.MainPage">
<controls:CustomWebView x:Name="webView" HorizontalOptions="FillAndExpand" VerticalOptions="FillAndExpand">
</controls:CustomWebView>
</ContentPage>
MyWebPage.xaml.cs
namespace XamWebView
{
// Learn more about making custom code visible in the Xamarin.Forms previewer
// by visiting https://aka.ms/xamarinforms-previewer
[DesignTimeVisible(false)]
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
webView.Source = "https://xamarinmonkeys.blogspot.com/";
}
}
}
Android
Note: Must add the following line in your webview renderer
global::Android.Webkit.WebView.SetWebContentsDebuggingEnabled(true);
Go to Chrome on your Machine then inspect any webpage. Then click three dots, then go to More tools > Remote Devices. Now you can see at the bottom device setting URL you can click that URL.
chrome://inspect/#devices
Run your app in Emulator
Now go to the webpage you able to see your webpage link then click inspect.
Finally, you can inspect your webview.
iOS
Note: Check your iOS simulator have web inspector option enable it.
Go to Settings > Safari > Advanced, and toggling the Web Inspector option on.
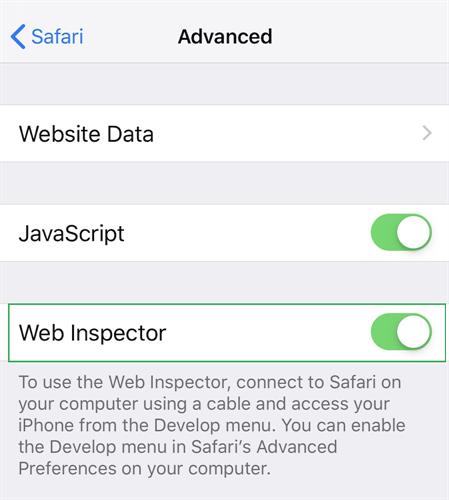
Next, you must also enable developer tools in Safari. Go to Safari > Preferences in the menu bar. Click on the Advanced tab and then enable the Show Develop menu option at the bottom.
Finally, Run your app the go-to Safari, Click on Develop in the menu bar and hover over the dropdown option that is your iOS device's name to show a list of webview instances running on your iOS device
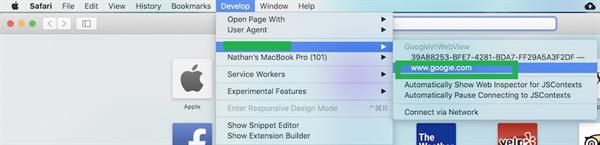
I hope you have understood how to enable debugging in webview in Xamarin.Forms.
Thanks for reading. Please share your comments and feedback. Happy Coding :)