Xamarin.Forms - Mobile Network Speed Check(Slow or Fast) in Android
In this article, you will learn how to check you mobile network speed(slow or speed) using android native in Xamarin.Forms.
Xamarin.Forms - Mobile Network Speed Check(Slow or Fast) in Android
Introduction
Xamarin.Forms code runs on multiple platforms - each of which has its own filesystem. This means that reading and writing files are most easily done using the native file APIs on each platform. Alternatively, embedded resources are a simpler solution to distribute data files with an app.
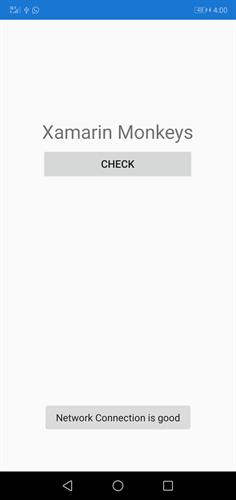
Prerequisites
- Visual Studio 2017 or later (Windows or Mac)
Setting up a Xamarin.Forms Project
Start by creating a new Xamarin.Forms project. You wíll learn more by going through the steps yourself.
Create a new or existing Xamarin forms(.Net standard) Project. With Android and iOS Platform.
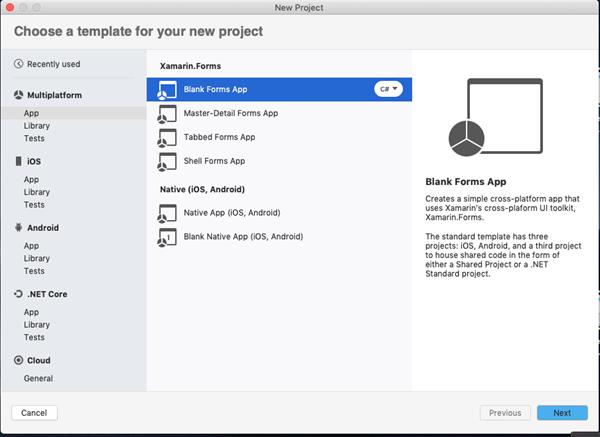
Create an Interface
public interface INetwork
{
bool IsConnected();
bool IsConnectedFast();
}
Create a network connectivity
The following code will check the Mobile network connection and Check the Network status whether speed or slow.
NetworkConnectivity.cs
public class NetworkConnectivity
{
public static NetworkInfo GetNetworkInfo(Context context)
{
ConnectivityManager cm = (ConnectivityManager)context.GetSystemService(Context.ConnectivityService);
return cm.ActiveNetworkInfo;
}
/**
* Check if there is any connectivity
* @param context
* @return
*/
public static bool IsConnected(Context context)
{
NetworkInfo info = NetworkConnectivity.GetNetworkInfo(context);
return (info != null && info.IsConnected);
}
/**
* Check if there is any connectivity to a Wifi network
* @param context
// @param type
* @return
*/
public static bool IsConnectedWifi(Context context)
{
NetworkInfo info = NetworkConnectivity.GetNetworkInfo(context);
return (info != null && info.IsConnected && info.Type == ConnectivityType.Wifi);
}
/**
* Check if there is any connectivity to a mobile network
* @param context
// @param type
* @return
*/
public static bool IsConnectedMobile(Context context)
{
NetworkInfo info = NetworkConnectivity.GetNetworkInfo(context);
return (info != null && info.IsConnected && info.Type == ConnectivityType.Mobile);
}
/**
* Check if there is fast connectivity
* @param context
* @return
*/
public static bool IsConnectedFast(Context context)
{
NetworkInfo info = NetworkConnectivity.GetNetworkInfo(context);
TelephonyManager tm = TelephonyManager.FromContext(context);
return (info != null && info.IsConnected && NetworkConnectivity.IsConnectionFast(info.Type, tm.NetworkType));
}
/**
* Check if the connection is fast
* @param type
* @param subType
* @return
*/
public static bool IsConnectionFast(ConnectivityType type, NetworkType subType)
{
if (type == ConnectivityType.Wifi)
{
return true;
}
else if (type == ConnectivityType.Mobile)
{
switch (subType)
{
//case TelephonyManager.NETWORK_TYPE_1xRTT:
case NetworkType.OneXrtt:
return false; // ~ 50-100 kbps
//case TelephonyManager.NETWORK_TYPE_CDMA:
case NetworkType.Cdma:
return false; // ~ 14-64 kbps
//case TelephonyManager.NETWORK_TYPE_EDGE:
case NetworkType.Edge:
return false; // ~ 50-100 kbps
//case TelephonyManager.NETWORK_TYPE_EVDO_0:
case NetworkType.Evdo0:
return true; // ~ 400-1000 kbps
//case TelephonyManager.NETWORK_TYPE_EVDO_A:
case NetworkType.EvdoA:
return true; // ~ 600-1400 kbps
//case TelephonyManager.NETWORK_TYPE_GPRS:
case NetworkType.Gprs:
return false; // ~ 100 kbps
//case TelephonyManager.NETWORK_TYPE_HSDPA:
case NetworkType.Hsdpa:
return true; // ~ 2-14 Mbps
//case TelephonyManager.NETWORK_TYPE_HSPA:
case NetworkType.Hspa:
return true; // ~ 700-1700 kbps
//case TelephonyManager.NETWORK_TYPE_HSUPA:
case NetworkType.Hsupa:
return true; // ~ 1-23 Mbps
//case TelephonyManager.NETWORK_TYPE_UMTS:
case NetworkType.Umts:
return true; // ~ 400-7000 kbps
/*
* Above API level 7, make sure to set android:targetSdkVersion
* to appropriate level to use these
*/
//case TelephonyManager.NETWORK_TYPE_EHRPD: // API level 11
case NetworkType.Ehrpd:
return true; // ~ 1-2 Mbps
//case TelephonyManager.NETWORK_TYPE_EVDO_B: // API level 9
case NetworkType.EvdoB:
return true; // ~ 5 Mbps
//case TelephonyManager.NETWORK_TYPE_HSPAP: // API level 13
case NetworkType.Hspap:
return true; // ~ 10-20 Mbps
//case TelephonyManager.NETWORK_TYPE_IDEN: // API level 8
case NetworkType.Iden:
return false; // ~25 kbps
//case TelephonyManager.NETWORK_TYPE_LTE: // API level 11
case NetworkType.Lte:
return true; // ~ 10+ Mbps
// Unknown
//case TelephonyManager.NETWORK_TYPE_UNKNOWN:
case NetworkType.Unknown:
return false;
default:
return false;
}
}
else
{
return false;
}
}
public static bool IsHostReachable(string host)
{
if (string.IsNullOrEmpty(host))
return false;
bool isReachable = true;
Thread thread = new Thread(() =>
{
try
{
//isReachable = InetAddress.GetByName(host).IsReachable(2000);
/*
* It's important to note that isReachable tries ICMP ping and then TCP echo (port 7).
* These are often closed down on HTTP servers.
* So a perfectly good working API with a web server on port 80 will be reported as unreachable
* if ICMP and TCP port 7 are filtered out!
*/
//if (!isReachable){
URL url = new URL("http://" + host);
URLConnection connection = url.OpenConnection();
//if(connection.ContentLength != -1){
//isReachable = true;
if (connection.ContentLength == -1)
{
isReachable = false;
}
//}
}
catch (UnknownHostException e)
{
isReachable = false;
}
catch (IOException e)
{
isReachable = false;
}
});
thread.Start();
return isReachable;
}
}
Android Implementation
Here, Implement the Interface and return the network status.
NetworkHelper.cs
[assembly: Xamarin.Forms.Dependency(typeof(NetworkHelper))]
namespace NetworkPOC.Droid
{
public class NetworkHelper : INetwork
{
Context context = Android.App.Application.Context;
public bool IsConnected()
{
return NetworkConnectivity.IsConnected(context);
}
public bool IsConnectedFast()
{
return NetworkConnectivity.IsConnectedFast(context);
}
}
}
Consume the NetworkHelper
Here, you will call the Network helper class and you will get the network speed.
MainPage.Xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
x:Class="NetworkPOC.MainPage">
<StackLayout HorizontalOptions="Center" VerticalOptions="Start" Margin="0,150,0,0">
<Label FontSize="Large" Text="Xamarin Monkeys"/>
<Button Text="Check" Clicked="Button_Clicked"></Button>
</StackLayout>
</ContentPage>
Here I show the result in a toast.
MainPage.xaml.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Xamarin.Forms;
using Xamarin.Essentials;
using System.Net.Http;
namespace NetworkPOC
{
// Learn more about making custom code visible in the Xamarin.Forms previewer
// by visiting https://aka.ms/xamarinforms-previewer
[DesignTimeVisible(false)]
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
var current = Connectivity.NetworkAccess;
if (current == NetworkAccess.Internet)
{
bool isConnectionFast = DependencyService.Get<INetwork>().IsConnectedFast();
if (isConnectionFast)
DependencyService.Get<IToast>().ShowToast("Network Connection is good");
else
DependencyService.Get<IToast>().ShowToast("Network Connection is slow");
}
else
{
DependencyService.Get<IToast>().ShowToast("No Internet Connection");
}
}
}
Run
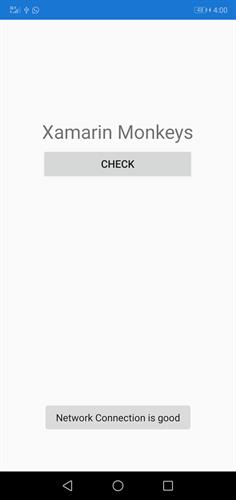
I hope you have understood you will learn how to check your mobile network speed(slow or speed) using an android native in Xamarin.Forms.
Thanks for reading. Please share your comments and feedback.
Happy Coding :)